Troubleshooting
Useful logs for Android
If you experience a crash on startup when running your game on Android, you might first want to get the crash reason. To do so, open a terminal and run the following command with your Android device connected to your computer:
adb logcat
Android and iOS
Application Crashes at Launch in Testflight or Shipping Builds
- Make sure you have the latest version of the plugin downloaded from the latest Engine version.
- Open your project's
Target.cs
and add the lines of code under theTODO
in the constructor:
// Your target. Already present in the file.
public class MyProjectTarget : TargetRules
{
public MyProjectTarget(TargetInfo Target) : base(Target)
{
Type = TargetType.Game;
ExtraModuleNames.AddRange( new string[] { "MyProject" } );
// TODO: Add these three lines:
if (Target.Platform == UnrealTargetPlatform.IOS)
{
GlobalDefinitions.Add("FORCE_ANSI_ALLOCATOR=1");
}
}
}
Build Failed: File google-services.json is missing.
If your google-services.json
is correctly placed but you get the following error while building.
> File google-services.json is missing. The Google Services Plugin cannot function without it.
UATHelper: Packaging (Android (ASTC)): Searched Location:
UATHelper: Packaging (Android (ASTC)): 5 actionable tasks: 5 executed
UATHelper: Packaging (Android (ASTC)): X:\AFSProject\app\src\release\google-services.json
UATHelper: Packaging (Android (ASTC)): X:\AFSProject\app\src\google-services.json
UATHelper: Packaging (Android (ASTC)): X:\AFSProject\app\google-services.json
Note that it searches for a project named AFSProject
and not for your own project name.
You can solve this error by disabling the plugin named AndroidFileServer
.
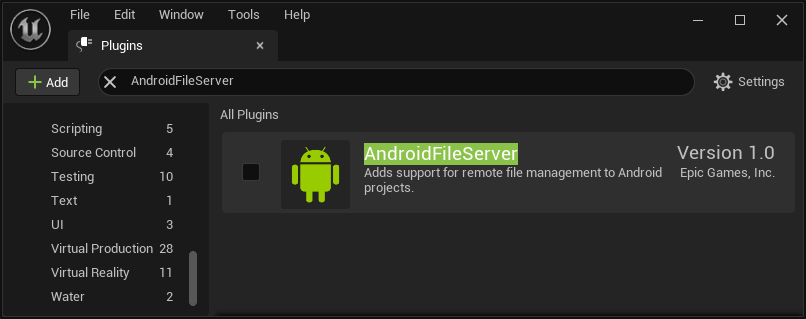
Failed to Sign In with Google: DEVELOPER_ERROR
- Missing SHA1 Fingerprint.
The most common cause of this issue is not having set the SHA1 fingerprint of the application in the Firebase Console. To solve the issue:
- Follow this guide to sign the Unreal Engine application.
- Get the SHA1 fingerprint of the app. This guide shows how to get the SHA1 fingerprint.
- Set the SHA1 for your Android app in the Firebase Console under project's settings.
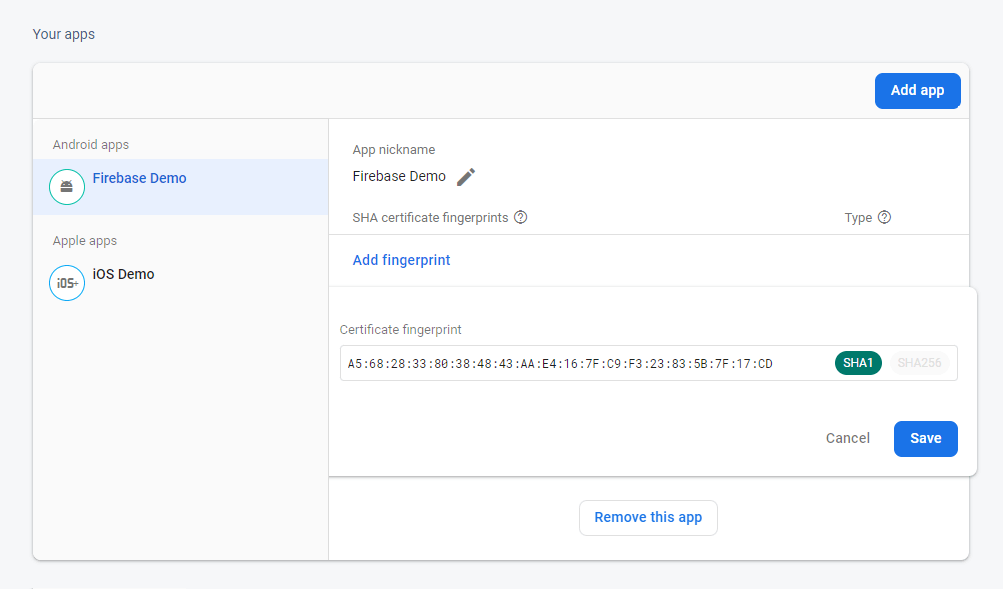
Invalid application ID
If the logs from adb
indicate Invalid application ID
, it means your AdMob application ID is invalid. The crash will disappear once you use the good one available on AdMob page.
The AdMob application ID is not an ad ID. It looks like ca-app-pub-XXXXXXXXXXXXXXXX~XXXXXXXXXX
.
Application crashes at startup
If your application crashes at startup after enabling the plugin, the reason is probably one of the following:
- Invalid
google-services.json
or invalidGoogleService-Info.plist
. - Invalid AdMob Application ID.
If you disabled AdMob for iOS without rebuilding the plugin, you still need a valid AdMob application ID. It is not required if you rebuilt the plugin.
Error: Cook failed. Editor terminated with exit code 16384
If you encounter this issue:
- Go in the Firebase Console. Click on
Realtime Database
in the left panel and create a new Realtime Database. - Download the
google-services.json
again and replace the one in your project with it.
uses-sdk:minSdkVersion x
cannot be smaller than version y
declared in library [com.google.firebase:firebase_messaging_cpp]
As we use the latest available libraries for Firebase C++ SDK, you might encounter this error if you target an old SDK version.
You need to change the minSdk to the y
value:
- Open Project's Config.
- Locate the
Platforms
>Android
>APK Packaging
>Minimum SDK Version (19=KitKat, 21=Lolipop)
config. - Set its value to
y
.
Packaging failed. Dependency resolved to an incompatible version
This error means that there is a plugin using Google's libraries of a different version, creating a conflict.
There are two options to solve the issue:
- Simply disable the other plugin.
- Change Firebase Features' or the other plugin's Google's libraries version. It can get complicated and requires some code changes. If you are facing this issue and want to use this solution, please contact us by email.
Undefined symbols when packaging for Android with architecture x86
or x86_64
.
For some Engine versions, the plugin only comes with the Firebase C++ SDK compiled for arm64-v8a
and armeabi-v7a
for Android.
To add support for x86 or x86_64:
- Download the binaries from the corresponding link x86_64 or x86.
- Unzip the files and copy them to
FirebaseFeatures/Source/ThirdParty/firebase_cpp_sdk/8.9.0/libs/android/{arch}
. i.e. forx86_64
, the following file has to exist:FirebaseFeatures/Source/ThirdParty/firebase_cpp_sdk/8.9.0/libs/android/x86_64/c++/libfirebase_app.a
. - Open
FirebaseFeatures/Source/FirebaseFeatures.Build.cs
and uncomment the line297
(x86) or298
(x86_64).
Desktop
Linux - libsecret-1.so
: cannot open shared object file.
The Linux Firebase SDK has a dependency on libsecret. You can install it with apt:
sudo apt-get install -y libsecret-1-0
Crashes when calling Firebase - Features
functions.
If you encouter crashes when using the Desktop platform, the cause is very likely a missing google-services.json
file. Follow the instructions here correctly. Make sure to check the Output Log after editor startup.
If you plan to only use Firebase - Features
on iOS, you'll have to download the .json
and the .plist
to test it in editor.
Crash in Packaged Game caused by Firestore
If the game crashes in packaged games with Firestore enabled, the cause is most likely Firestore's persistence system. It can be disabled in the plugin's settings as shown in the below image.
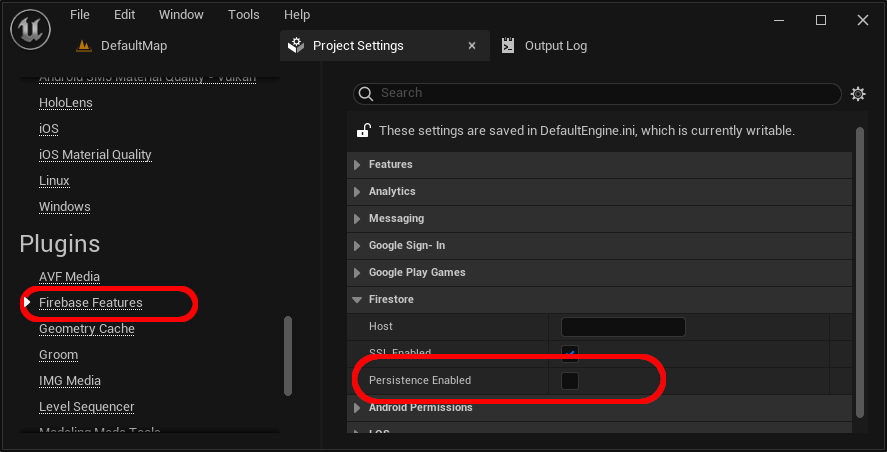
Linux - Build fails with duplicate symbols for libcurl
- Open
Plugins/FirebaseFeatures/Source/ThirdParty/firebase_cpp_sdk/libs/linux/x86_64_PIC
. - Delete the file
libcurl.a
.