Cloud Functions
Cloud Functions for Firebase is a serverless framework that lets you automatically run backend code in response to events triggered by Firebase features and HTTPS requests. Your JavaScript or TypeScript code is stored in Google's cloud and runs in a managed environment. There's no need to manage and scale your own servers.
Call a function
To call a Cloud Function, you have to first retrieve an Https Callable Reference with the node Get Https Callble
.
Once the reference is retrieved, you can call it with a Variant data parameter.
BlueprintsC++
#include "Functions/FunctionsLibrary.h"
#include "Functions/CallableReference.h"
/* First, we get the Cloud Function. */
static FFirebaseHttpsCallableReference Callable = UFirebaseFunctionsLibrary::GetHttpsCallable("my_function");
/* Calls the Cloud Function. */
Callable
(
/* The function's parameters */
FFirebaseVariant
({
{ TEXT("MyInt"), 12 },
{ TEXT("MyString"), TEXT("Value") }
}),
/* Our callback called after the function's execution. */
FFunctionsCallCallback::CreateLambda([](const EFirebaseFunctionsError Error, const FFirebaseVariant& Result) -> void
{
if (Error == EFirebaseFunctionsError::None)
{
// Function called.
}
else
{
// Failed to call the function.
}
})
);
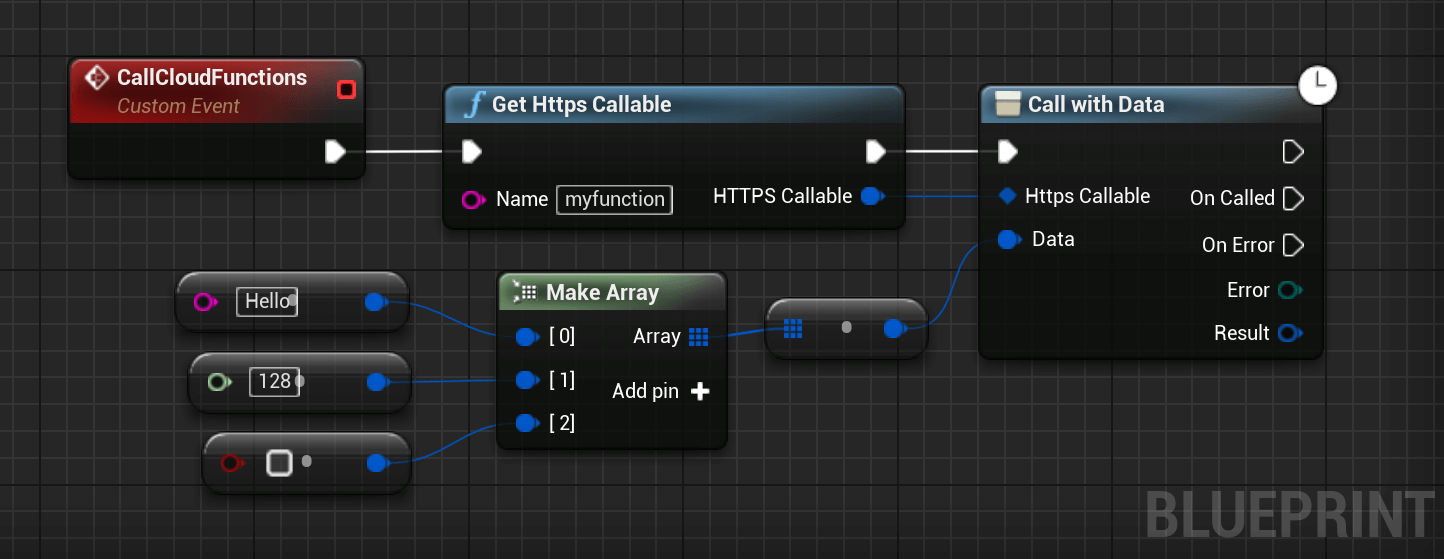
Write a Function
To learn more about writing Cloud Functions and how to deploy them, refer to the official Firebase Documentation.