AdMob
This section covers how to use AdMob with the Firebase - Features plugin.
AdMob helps you monetize your mobile app through in-app advertising. Three types of advertising are available.
For development, you should pick a Sample ad unit ID or your AdMob account might be flagged.
The examples require the plugin version 1.7.24 or newer.
Banner View
Banners are rectangular ads that occupy a portion of an app's layout. They can be refreshed automatically after a period of time.
// C++ example not available yet.
It is possible to easily show a banner using a helper node to create, load, and show a banner view.
Make sure to keep the banner in a variable so it doesn't get garbage collected and removed from the screen.
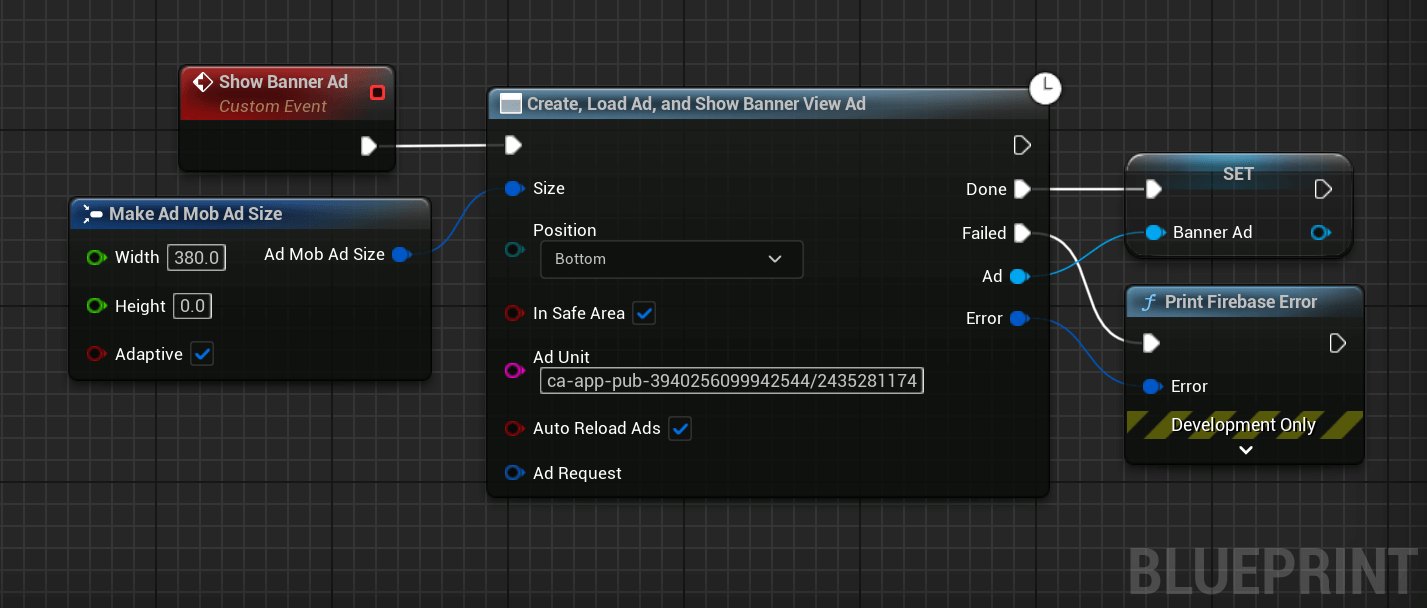
More methods are available to manage the banner:
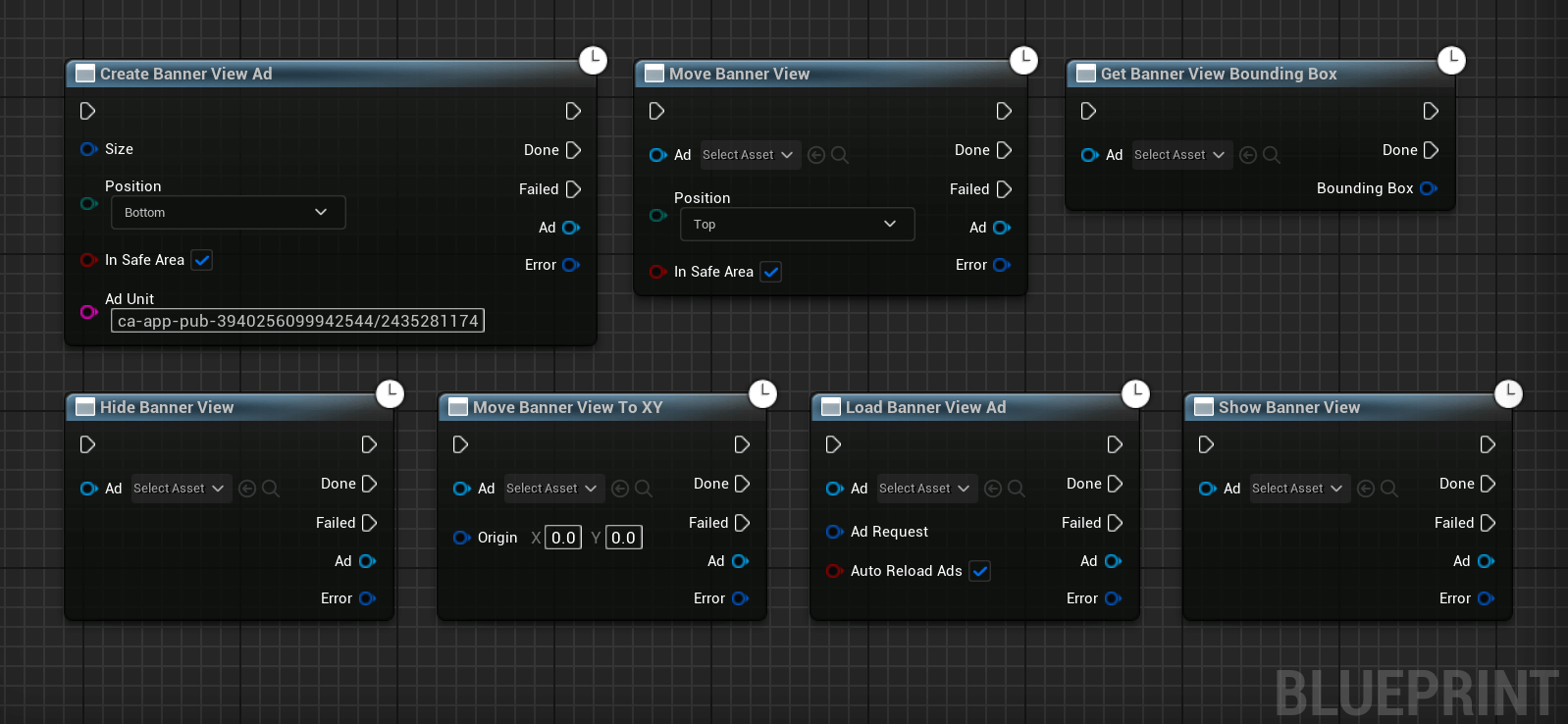
And it is possible to listen for multiple events emitted by the banner:
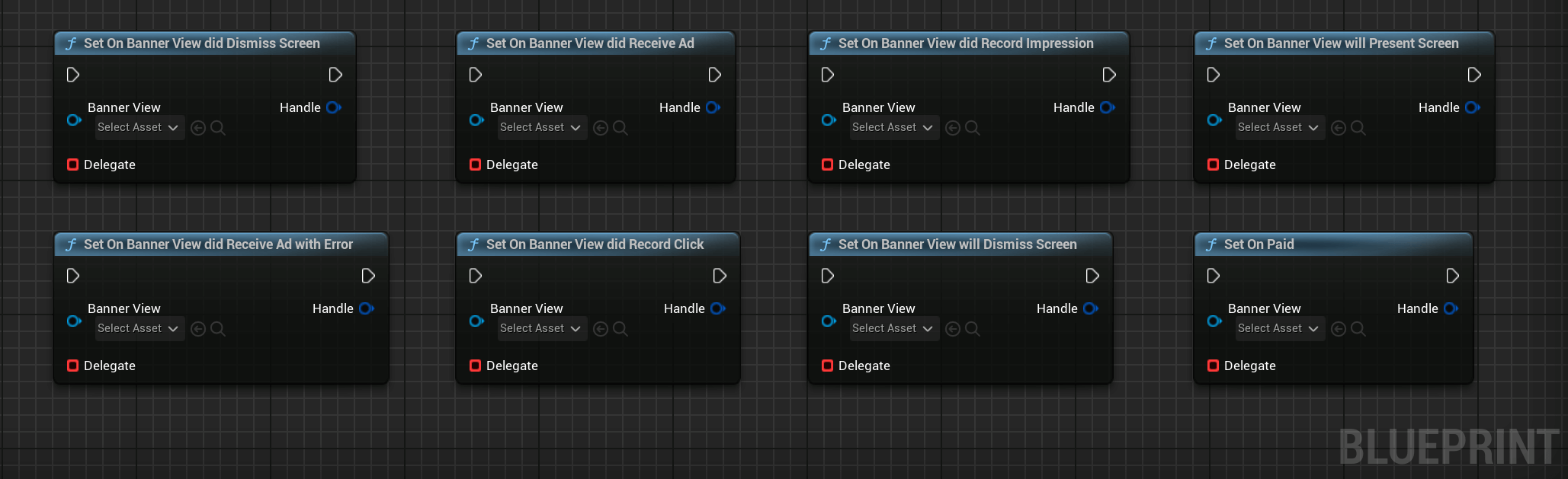
Interstitial Ads
Interstitial ads are full-page ad format that appear at natural breaks and transitions, such as level completion.
// C++ example not available yet.
The following Blueprint code can be used to show an interstitial ad using a helper node:
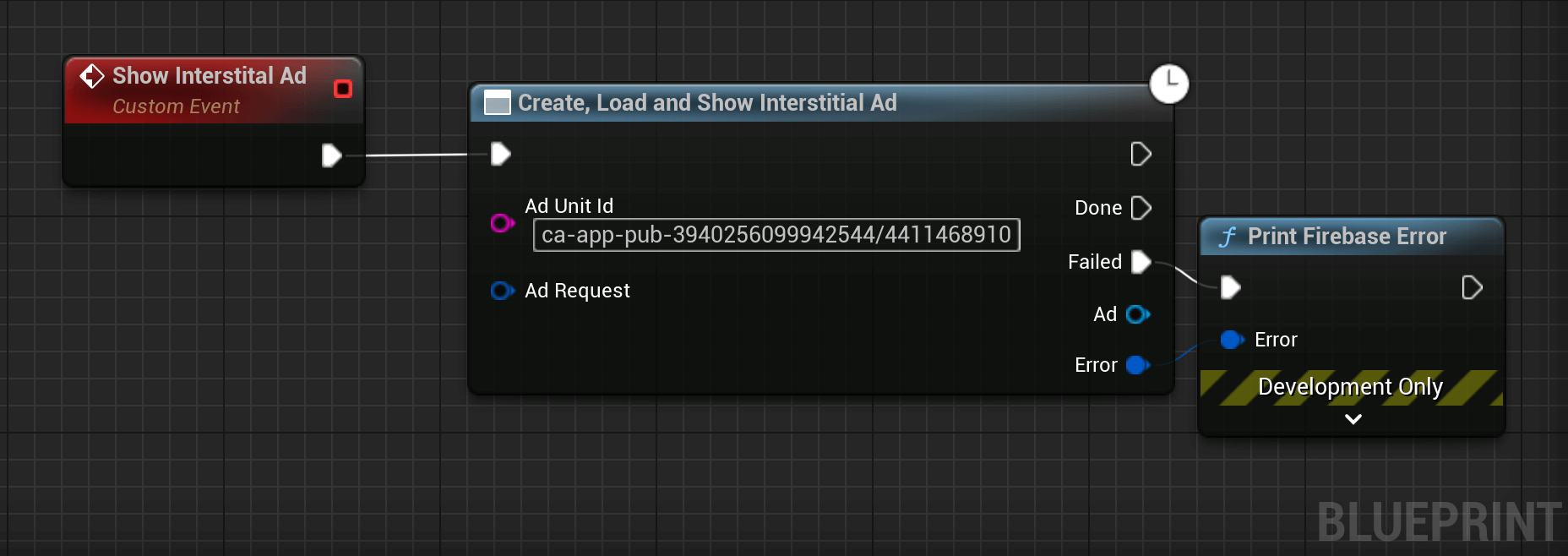
It is also possible to call each step separately, to preload ads for example:
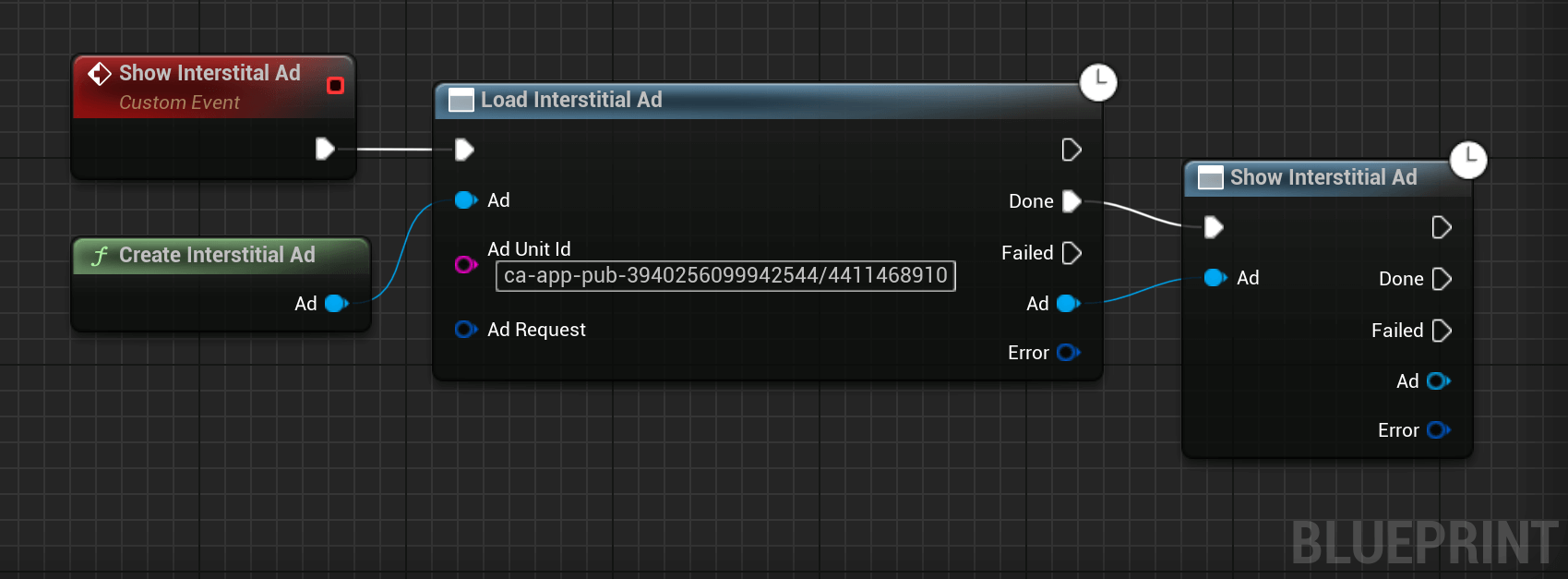
Multiple events are also available:
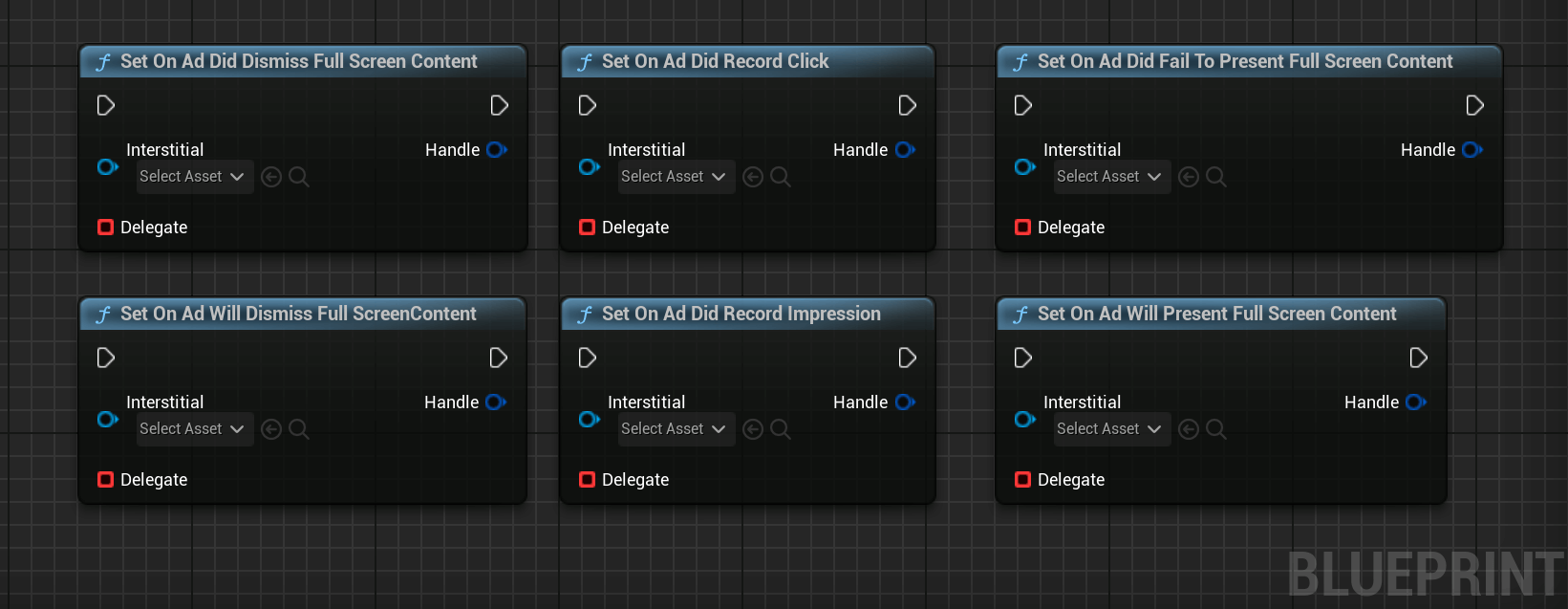
Rewarded Videos
Rewarded videos are ad formats that reward users for watching ads. They are great for monetising free-to-play users.
// C++ example not available yet.
Here's how to show a Rewarded Video to your users:
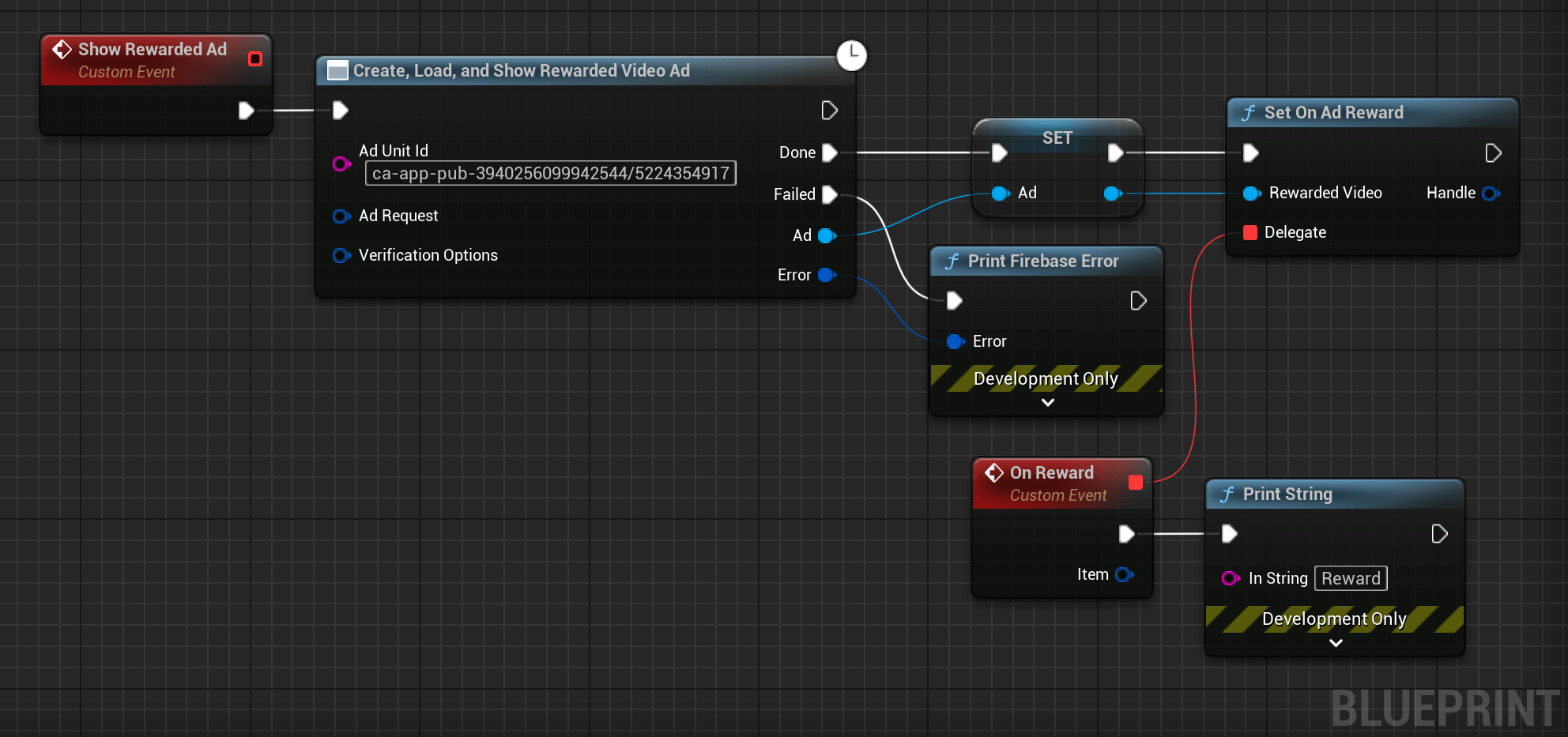
As Rewarded Videos take time to load, it is better to preload them before. The code can be modified to load an ad before showing it:
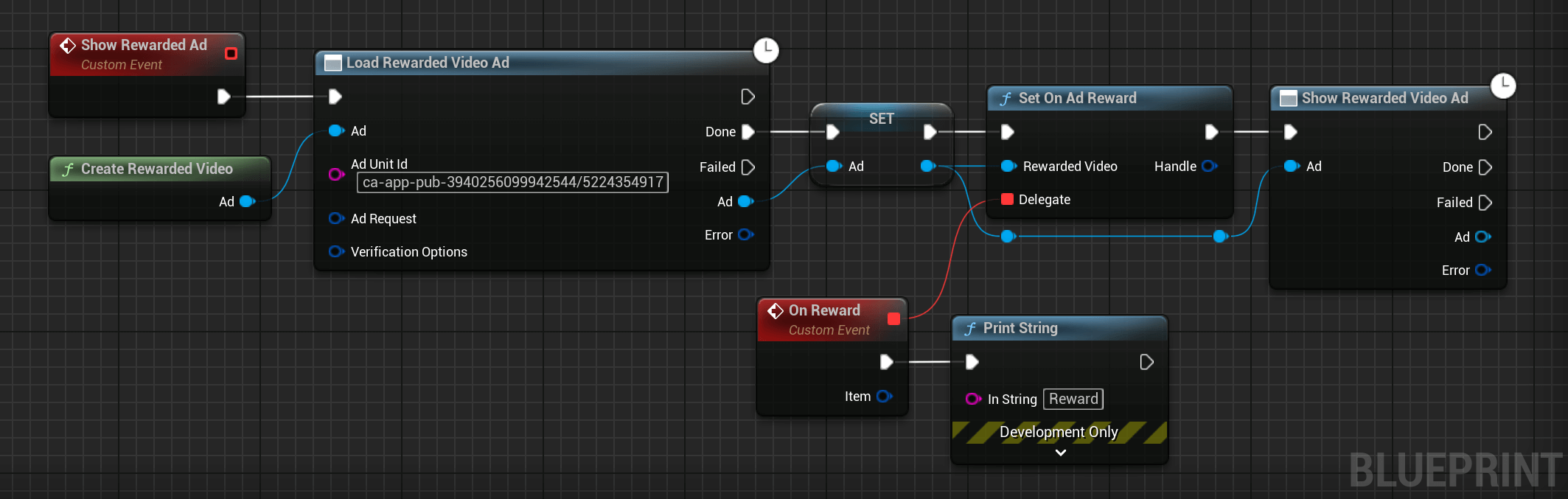
AppOpen Ads
App open ads are a special ad format intended for publishers wishing to monetize their app load screens. App open ads can be closed at any time, and are designed to be shown when your users bring your app to the foreground.
// File containing the UAppOpenAd class.
#include "AdMob/FbAppOpenAd.h"
/***********************************************
Create an ad.
************************************************/
UAppOpenAd* Ad = NewObject<UAppOpenAd>();
/***********************************************
Later, load an ad.
************************************************/
// Create the ad request.
FAdMobAdRequest Request;
Request.Keywords = { TEXT("game"), TEXT("fun") };
// Launch the load of the ad.
Ad->Load(
/* Ad Unit ID */
TEXT("ca-app-pub-3940256099942544/5575463023"),
/* Our request */
Request,
/* Callback when the ad is loaded. */
FFirebaseAdMobCallback::CreateLambda([](FFirebaseError Error)
{
if (Error)
{
// Failed to load the ad as something went wrong. Check the output log for the reason.
UE_LOG(LogTemp, Error, TEXT("Failed to load an ad: %s"), *Error.Message);
}
else
{
// Ad is loaded and ready to be shown.
}
})
);
/***********************************************
Finally, after the ad was loaded, show the ad.
************************************************/
/* Shows the ad */
Ad->Show(FFirebaseAdMobCallback::CreateLambda([](FFirebaseError Error)
{
if (Error)
{
// Failed to show the ad as something went wrong. Check the output log for reason.
UE_LOG(LogTemp, Error, TEXT("Failed to show ad: %s"), *Error.Message);
}
else
{
// Ad is currently on screen.
}
});
A helper method can be used to quickly implement AppOpen ads:
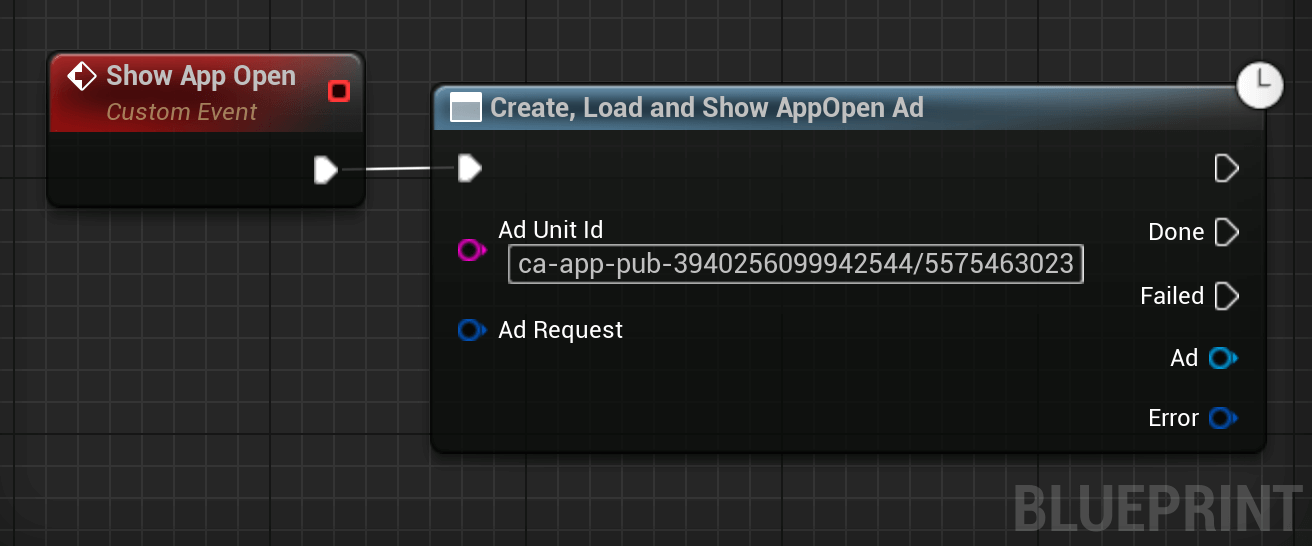
It is also possible to call the three functions separately: New App Open
, Load Ad
, and Show Ad
to be able to pre-load ads.
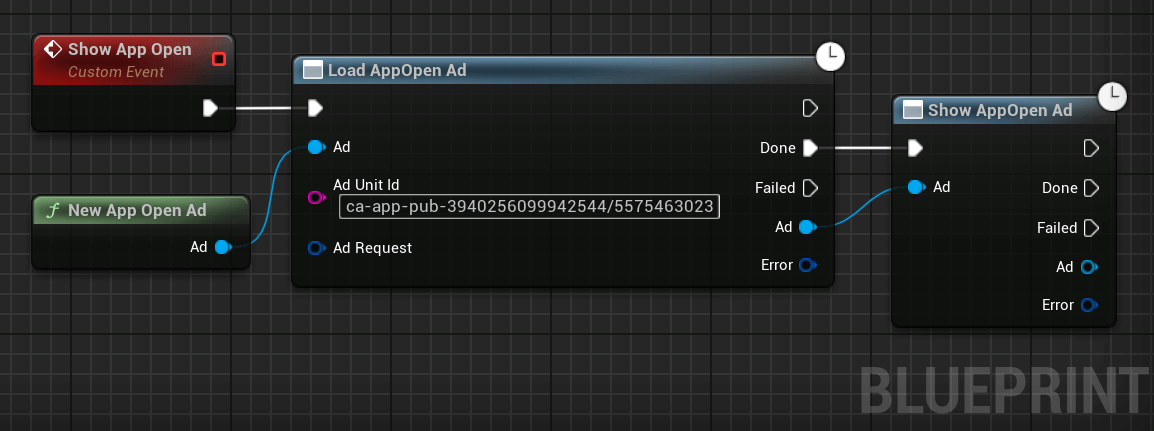